Discussion
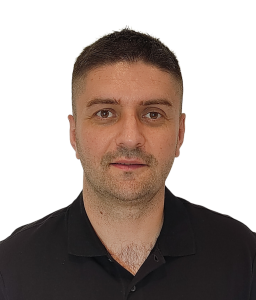
Pegasystems Inc.
PL
Last activity: 2 Apr 2025 5:34 EDT
Getting started with SDKs
This article is part of series of articles named Constellation Flexible UI. Please check table of contents: https://support.pega.com/discussion/constellation-flexible-ui
Previous article was an introductory one showing all possible UI choices offered by Constellation architecture. This time we will dive deep into custom UI offering made possible by Constellation SDKs. As told already, it is not the only one option for building custom UI with Constellation. But it is the default one that is believed to cover most of the clients needs.
SDK stands for Software Development Kit. It's a set of tools, libraries, documentation, and code samples that developers use to create software applications for a specific platform, system, or framework. SDKs provide developers with everything they need to build applications that can interact with the platform or system they're targeting, such as APIs, debugging tools, and compilers. This definition pretty much well describes Constellation SDKs, with some small exceptions.
To benefit fully from this series of articles I assume that you are skillful with Pega. It is advised to go through Constellation adoption mission to understand more about Constellation
Please note what is supported by Constellation SDKs:
- Embedding in another customer Portal or Application
- Pega’s web self-service portal
- Customer Service tasks
While we can do a lot with SDKs there are several limitations that we shall remember of. SDKs are primary targeted for Web Self Service solutions. SDKs don't support:
- Customer Service Desktop
- Full Customer Service Interaction lifecycle
- Explore Data - Insights
- Advanced widgets for Case Workers
Adopting Best Practices
When utilizing Constellation SDKs and APIs, it's essential to follow best practices for maintainability, performance, and security:
- Prioritize the use of out-of-the-box Constellation templates and patterns before creating custom extensions or integrations
- Adhere to Pega's recommended development practices and guidelines for Constellation customizations
- Implement proper error handling, logging, and monitoring mechanisms for custom integrations and extensions
- Follow security best practices, such as input validation, authentication, and authorization, when interacting with Pega Platform data and services.
We will refer to those best practices in next articles.
Preparation for SDK implementation
To fully understand mechanics of SDKs following skills are required:
- Java Script, ES6 preferred
- React, Angular of Web components
- Type Script Java Script tooling (NVM, Node.js, NPM, Linter, Prettier etc)
Here are great resources to get you started with React Check out this great course on Coursera straight from Meta: https://www.coursera.org/learn/react-basics
It is important here to mention technologies supported by SDKs, which are:
- React
- Angular
- Web Components
Level of feature support in given SDK might be different. Please refer to documentation specific to your SDK.
Our discussions and learnings of SDKs will be based on public documentation available here:
- https://docs.pega.com/bundle/constellation-sdk/page/constellation-sdks/sdks/constellation-sdks.html
- https://docs.pega.com/bundle/pcore-pconnect/page/pcore-pconnect-public-apis/api/using-pcore-pconnect-public-apis.html
Those two doc bundles are separated from each other and from main Pega documentation this is why it is worth to keep those two links handy.
It is worth to go through the documentation and make yourself familiar with it before proceeding with configurations described in this tutorial.
Another resources that might be helpful when developing with SDKs is documentation of Constellation Design System: https://design.pega.com/. It describes basics concepts and principles of design system as well as shows sample code explaining how to use out of the box components.
Sample application
This series of articles aim to provide practical information on how to use Constellation SDKs. Following the articles you will be able build sample portal that integrates with SDKs. You can see here a demo of portal that we will build: https://www.youtube.com/watch? v=LrdKmpy3QCU.
It looks pretty neat, doesn't it? Under the hood there is old good Pega. Could you achieve similar results with UI Kit? I don't think so. I believe that power and flexibility of new architecture is apparent for you.
Here is source code of demoed portal: https://github.com/kamiljaneczek/Sweet-Life-Pega. Take a look into Package folder where you can find Tell us More application, reference Pega application. To proceed with hands-on exercises please import this application into Infinity 24 server.
We will investigate it in more details when we start implementation of SDKs.
How SDKs work?
We saw final solution, but let's take a step back (a few steps back in fact) and talk in more details how the SDKs work.
When looking on diagram below we have couple immediate observations:
- there is a responsibility split between Pega and clients - some components are Pega managed, some (like Alternate DX component) are in capable hands of clients
- a lot of things happen on device, rather than on server. This paradigm is called Client Orchestration and is true with all modern frameworks. In a nutshell and on high level. JSON metadata with data describing UI configuration is sent via DX API to client and JS code residing on client side is responsible for hydrating the final HTML code. Exactly thanks to this feature we are able to customize look and feel of of UI
- third one, a little bit less obvious is that custom portals use the same orchestration layer as ooth Constellation portal which is Constellation orchestration layer
- fourth, also not visible on first sight. It's important to remember that regardless of the chosen approach or portal, developers will still need to perform the UI Authoring of the views within the Pega Platform. Fronted end portal will use metadata coming from View definitions to render the UI in a way that depends on fronted implementation. But the Views must be created so we can fetch their definition.
The interesting part from SDK point of view is the part with the Alternative Design system block which includes:
- alternative presentation components - overridden version of default components with alternative design system applied
- alternative DX components - custom DX component with alternate design system
- SDKs - which is abstraction layer and set of functions and tools that ease the work of connecting to Infinity with Constellation Orchestration layer, the public JS API also known as ConstellationJS. SDKs do the heavy lifting of:
- authentication on Infinity server requesting resources
- translating ootb components to their overridden versions
- The foundation of communication between the client, the Device, and the Infinity is DX API. Fortunately thanks to Constellation JS and SDKs, usage of DX API (raw HTTP requests) is abstracted for us and we operate on higher level functions (the utilized DX API endpoints)
BTW, There is no possibility to host custom portal on Pega Cloud. Hosting custom portal is client responsibility.
Let's get started
Well, getting started with SDKs can be intimidating, unless you have 10 years experience with Pega and you are skillful JS developer. While this might be true typically will not be. Worry not, this series of articles is meant to bridge the knowledge gap and allow you to be proficient with Pega SDKs.
I will collect here information on how to reach a basic level of understanding of SDKs, that will allow you to:
- understand how SDKs can be utilized to build custom UI solutions
- help customers deciding on an implementation approach
- modify sample MediaCo application to run customer-specific Case Type and rebrand UI to match customer needs
- remove dependency to Material UI and implement customer Design System
React SDK
Let's start investigation with what is inside React SDK, and first of all where to find it.
First place to visit is Pega Marketplace, where you can download SDK: https://community.pega.com/marketplace/component/react-sdk. Be sure that you using SDK version matching version of your Infinity server. SDK-R v23.1.11 only compatible with Pega Infinity '23 and is related to the release/23.1.1 branch of the React SDK repository.
Let's see what SDK includes:
- ConstellationJS Engine to React bridge - basically this is the code that ease connectivity to Constellation JS engine by providing the abstraction layer and hiding some of the complexity of ConstellationJS API
- Sample integration of the React bridge with the Material UI design system - this is sample implementation of Material UI Design Sytle, to show the capabilities of SDKs to perform custom styling
- DX components - DX components implementing Material UI overriding default
- Constellation components
- MediaCo - it is a sample Pega application
- Documentation: SDK Guide, ConstellationJS Engine overview, component building instructions, and ConstellationJS API documentation
When downloaded, inside you will find documentation as well as RAP for sample application MediaCo.
MediaCo application provided by Pega is only a sample application to get you started with SDKs. By no means it shall be treated as production ready application.
Source code of SDK can be found in this Github repo: https://github.com/pegasystems/reactsdk
Download code locally from repo or fork it to prepare for development.
Similar structure we will find for other technologies: Angular and Web Components
Angular
Here are links to assets for Angular https://github.com/pegasystems/angular-sdk
https://community.pega.com/marketplace/component/angular-sdk
Web components
Here are links to assets for Web components:
https://github.com/pegasystems/web-components-sdk
https://community.pega.com/marketplace/components/web-components-sdk
Installation
After downloading sample application install it on Infinity server following instructions from documentation: https://docs.pega.com/bundle/constellation-sdk/page/constellationsdks/sdks/verifying-pega-infinity-dev-server.html. Second step involves opening content of starter pack repo in IDE of your choice and running install command:
npm install
which will install all the SDK dependencies listed in package.json file . Make sure you use correct version of packages: https://docs.pega.com/bundle/constellation-sdk/page/constellationsdks/sdks/configuring-package-json-file.html
Up until now all steps were framework agnostic. Since now procedure will be different for each of SDKs. Next articles in this series will be based on React SDKs but with some minor adjustments it shall be applicable also for other technologies.