Discussion
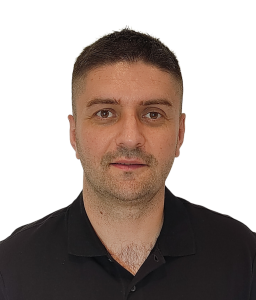
Pegasystems Inc.
PL
Last activity: 17 Oct 2024 2:58 EDT
React SDK
This article is part of series of articles named Constellation Flexible UI. Please check table of contents: https://support.pega.com/discussion/constellation-flexible-ui
In previous articles we learned about custom UI options in Pega and we talked about the best way to interact with ConstellationJS which are Constellation SDKs. This article goes further to understand more details about SDKs. As example in this and further articles we will use React SDK.
React is a popular JavaScript library used for building user interfaces, particularly for web applications. Developed by Facebook, React is known for its efficiency and flexibility in creating interactive and dynamic UI components. React is very popular so it is good choice to explain how to use React with Constellation. It is believed that most of clients of Pega would like to use either React or Angular.
BTW, technically React is library not a full framework!
Configuration of SDK
Follow instructions in official documentation: https://docs.pega.com/bundle/constellation-sdk/page/constellation-sdks/sdks/configuring-sdk-config-json.html to configure SDK. Steps all clearly described so not much comments are needed.
Most important part of SDK configuration is to configure authorization. For testing we can use OAuth2 authentication the way it is described in official documentation. While for testing this is good enough, this is not a production ready solution. We would like to avoid login popup and maybe find some way for automatically and seamless login. We will implement kind of a anonymous login in one of next articles.
Sample config of mashup:
Note: sdk-config.json is standard JSON file and JSON format doesn't support comments. This is why additional fields (not used by logic) are present in config file, example: field named mashupClient_comment3
Running sample application
Code delivered with SDKs is a functional application supporting two modes:
- embed, Pega UI embedded into external
- poral full portal
It is believed that embed mode is more popular choice so will concentrate on it. Below please find screenshot from Portal mode, where whole portal along with menu bar on left is rendered.
The Embedded use case is the most common use of the React SDK. The Embedded use case presents a Pega flow action running inside a non-Pega web application. Embed mode can also render a Landing page from Pega. This is a powerful concept as it gives a lot of flexibility with exposing UI from Pega and embed it on external portal. The Portal use case presents the Pega portal UI for a Constellation-based Pega application.
Note: Use portal or embed address without .html as it is not configured be default in list of redirect URIs in OAuth2 config in Pega
Due to complex nature of tasks that we would like to perform, SDKs are not only a set of functions and tools but it also come with prepackaged application. This is not typical for SDKs and is rather present in starter packs.
It has both pluses and downsides. For the pluses we can include:
- you can run application just after installation and with very few steps of configuration. Otherwise you would need to spent a lot of time before you see any result on a screen you got sample code to get you started with development very fast
- you can see example of how to implement alternate design system on a example of Material UI design system
For downsides:
- it is difficult to distinguish what is SDKs and what is part of sample implementation sample application is not a production grade one it is just an example
- it is time consuming to remove all dependencies to Materials UI and you need to have a little bit of understanding of Material UI to know why some components are implemented way they are
- only one way starting with SDKs is to start with repo, there is no possibility just to add SDKs are dependency which might be significant limitations, ie for monorepo architecture
Anatomy of SDK
As mentioned, SDKs include sample app which refers several npm packages in package.json file. Let's see what is what using React SDK example.
Generic NPM packages
- https://www.npmjs.com/package/@pega/constellationjs - ConstellationJS, the orchestration layer. It is the abstraction layer that allows us for easy connectivity to Infinity over DX API abstracting the the need to use raw fetch requests. Note: Constellation JavaScript Engine - is not open sources, it is Pega propriety code
- https://www.npmjs.com/package/@pega/pcore-pconnect-typedefs - package with TypeScript types for PCore and PConn objects that we will use extensively. Pega SDKs strives to be full type safe (but we are not quite there)
- https://www.npmjs.com/package/@pega/auth - package that implement popular auth methods like oAuth2. Why implementing it on your own when you can use this library?
- https://www.npmjs.com/package/@pega/configs - cumulative package with JS tooling like eslint, prettier etc. This is not mandatory package but advised. You are fine to update the config to match your coding preference
React specific packages
There are also technology specific packages. Here is example for React:
https://www.npmjs.com/package/@pega/react-sdk-components - this package comprise two modules:
- bridge - the code that allows to easily create PCore and PConn object as well as use Component Map to load our specific component implemented with custom DS rather than the default one from SDK (based on Material UI)
- components - components that are part of SDKs. Sample implementation using Material UI that overrides Constellation components
- https://www.npmjs.com/package/@pega/react-sdk-overrides - This Node package comprises React components that are designed to be overridden by the React SDK
- https://www.npmjs.com/package/@pega/cosmos-react-core
- https://www.npmjs.com/package/@pega/cosmos-react-work
Please find below packaged specific to Angular and Web Components:
- https://www.npmjs.com/package/@pega/angular-sdk-overrides
- https://www.npmjs.com/package/@pega/angular-sdk-components
Technicalities
This chapter lists prerequisites to run React SDK. Make sure you fulfill all prerequisites, otherwise you might encounter issues
Prerequisites:
- node 18.12.1/18.13.0
- npm 8.19.2/8.19.3 - do not use npm 9 or npm 10
Dependencies:
Below SDKs dependencies are listed (to React libraries.)
- "react": "^17.0.2",
- "react-dom": "^17.0.2",
- "react-redux": "^7.2.4",
- "react-router-dom": "^5.3.4",
Please make sure you use proper versions, otherwise you might encounter issues.
If you would like to integrate SDK inside external portal that uses React 18 you might find it problematic. In case you can not solve dependency issues connecting to Constellation without SDK might be only one option.