Question
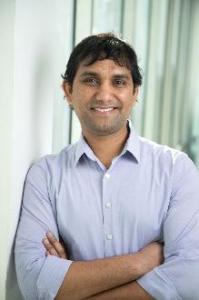
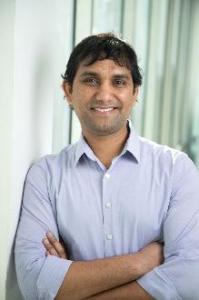
BlueCross BlueShield of NC
US
Last activity: 26 Mar 2021 15:38 EDT
Unzip files and attach to case
Hello,
We have requirement
1) To create cases for every zip file created on local server. (Nightly batch job loads zip files to Pega server)
2) Unzip the folder and attach all the files in the zip folder to created case.
I am thinking to write custom java code to unzip the folder. I am stuck on how to attach the files to case after unzipping.
Any help is greatly appreciated.
-
Like (0)
-
Share this page Facebook Twitter LinkedIn Email Copying... Copied!
Accepted Solution
Updated: 26 Mar 2021 15:38 EDT
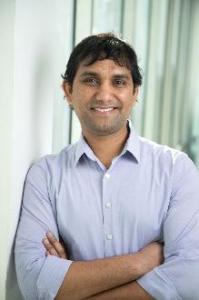
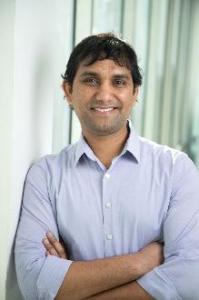
BlueCross BlueShield of NC
US
Sorry for the delay. This is resolved.
I unzipped the files using below java code and than used pxUploadAndAttachFile activity to attach files to the case. The pre-req to call pxUploadAndAttachFile activity is to move the file to serviceexport directory using java.
Code :
java.io.File path = new java.io.File(unzip);
Sorry for the delay. This is resolved.
I unzipped the files using below java code and than used pxUploadAndAttachFile activity to attach files to the case. The pre-req to call pxUploadAndAttachFile activity is to move the file to serviceexport directory using java.
Code :
java.io.File path = new java.io.File(unzip);
java.io.File [] files = path.listFiles(); for (int i = 0; i < files.length; i++){ if (files[i].isFile()){ String fileName = files[i].getName(); String destFile = serviceexport + fileName; oLog.info("copying file : " +fileName); java.io.File source = new java.io.File(unzip+"/"+fileName); java.io.File dest = new java.io.File(destFile); source.setReadable(true); source.setWritable(true,false); source.setExecutable(true,false); try { java.nio.file.Files.copy(source.toPath(), dest.toPath()); oLog.info("Copied"); } catch (java.io.IOException E) { oLog.infoForced("Error"+ E.getMessage()); E.printStackTrace(); } //upload process ParameterPage newParamsPage = new ParameterPage(); newParamsPage.putString("hiddenFileName", fileName); String activityClass = "@baseclass"; String activityName = "pxUploadAndAttachFile"; HashStringMap params = new HashStringMap(); params.putString("pxObjClass", "Rule-Obj-Activity"); params.putString("pyClassName", activityClass); params.putString("pyActivityName", activityName); tools.doActivity(params, myStepPage, newParamsPage); oLog.info("uploaded"); } }
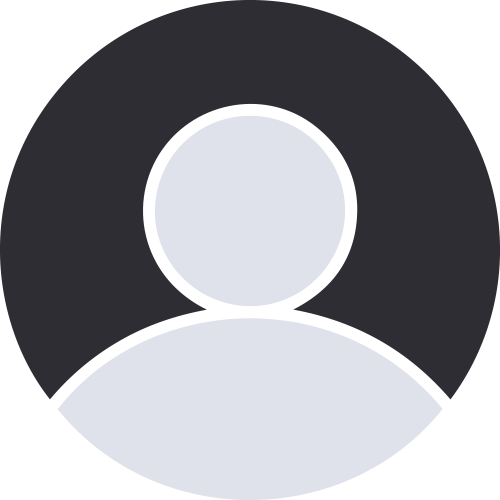
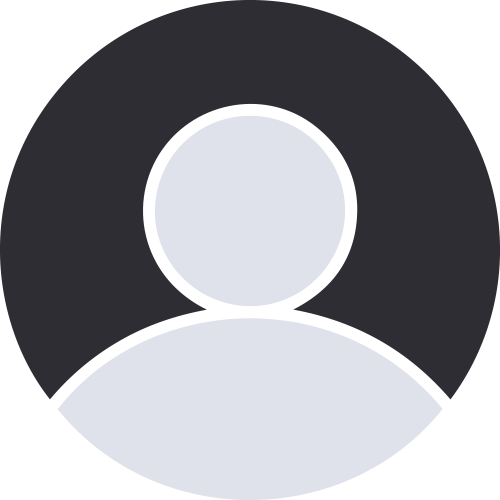
Pegasystems Inc.
FR
Is it not possible to unzip outside Pega and then just use a FileListener to attach those files? What platform version is it?
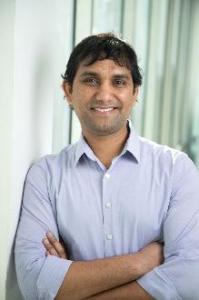
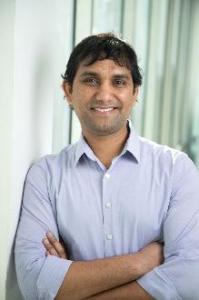
BlueCross BlueShield of NC
US
we can but we have to create case for every zip file landing on Pega server. That's the reason I was thinking to write java to iterate over zip files and create case in the loop.
We can't use file listener because the zip is a complex structure it contains multi folder with multi files with multiple formats. We have to take all files from all folders and attach to a case.
We are on Pega 8.2.7
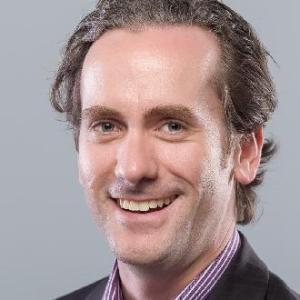
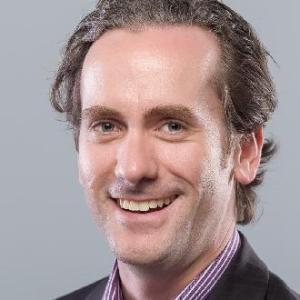
Pegasystems Inc.
AU
I would recommend the following:
Run the Tracer whilst attaching a file through the end user portal to understand what rules are involved in attaching a file to a case. Alternatively, investigate the Pega API endpoint /attachments/upload (assuming it is available on your Platform version, check the "Pega API" help menu option in Dev Studio). Your implementation might even choose to call this API to fulfil the attachment operation.
If your attachments are being stored in web storage or a Repository (as opposed to in the Pega database) then you will want to reuse the rules that know how to orchestrate the appropriate API calls to where the attachments are stored, based on how the application rule is configured. The typical outcome is that Pega stores an instance of Data-WorkAttach-File and Link-Attachment for each attachment.
I would recommend the following:
Run the Tracer whilst attaching a file through the end user portal to understand what rules are involved in attaching a file to a case. Alternatively, investigate the Pega API endpoint /attachments/upload (assuming it is available on your Platform version, check the "Pega API" help menu option in Dev Studio). Your implementation might even choose to call this API to fulfil the attachment operation.
If your attachments are being stored in web storage or a Repository (as opposed to in the Pega database) then you will want to reuse the rules that know how to orchestrate the appropriate API calls to where the attachments are stored, based on how the application rule is configured. The typical outcome is that Pega stores an instance of Data-WorkAttach-File and Link-Attachment for each attachment.
- If attachment content is stored in Pega, it is stored in Base64-encoded form in the pyAttachStream property of the Data-WorkAttach-File instance.
- If attachment content is stored in Web Storage or Repositories, a reference to the location in the remote storage system (a file path or a URL) is stored in the Data-WorkAttach-File instance.
With some Google searching you will be able to find some Java code that can unzip an archive. Ideally, the unzipping would be done into memory since you will either copy each streamed file either into a Data-WorkAttach-File clipboard page; or into the request of the /attachments/upload API call. Keeping it in thread-scoped memory reduces the risk of not cleaning up the file system, running out of disk space - or worse - a race condition where ZIP files containing the files of the same name get incorrectly attached to the wrong cases.
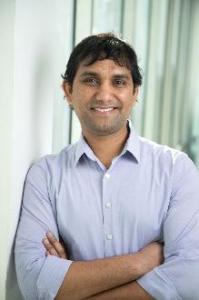
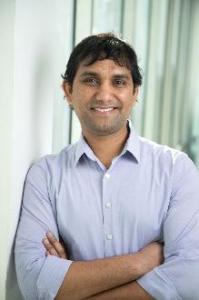
BlueCross BlueShield of NC
US
thank you. I was able to Unzip the file using Java.
I was looking for working model how to attach files to case outside of user portal.
Yep I am planning to trace the rules that gets triggered from user portal. Will check and keep you posted.
Thank You
Accepted Solution
Updated: 26 Mar 2021 15:38 EDT
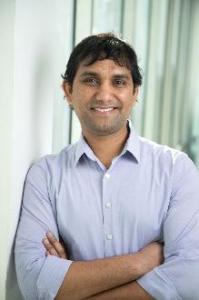
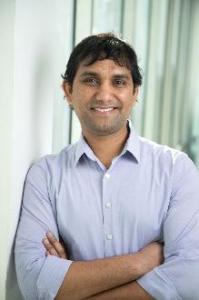
BlueCross BlueShield of NC
US
Sorry for the delay. This is resolved.
I unzipped the files using below java code and than used pxUploadAndAttachFile activity to attach files to the case. The pre-req to call pxUploadAndAttachFile activity is to move the file to serviceexport directory using java.
Code :
java.io.File path = new java.io.File(unzip);
Sorry for the delay. This is resolved.
I unzipped the files using below java code and than used pxUploadAndAttachFile activity to attach files to the case. The pre-req to call pxUploadAndAttachFile activity is to move the file to serviceexport directory using java.
Code :
java.io.File path = new java.io.File(unzip);
java.io.File [] files = path.listFiles(); for (int i = 0; i < files.length; i++){ if (files[i].isFile()){ String fileName = files[i].getName(); String destFile = serviceexport + fileName; oLog.info("copying file : " +fileName); java.io.File source = new java.io.File(unzip+"/"+fileName); java.io.File dest = new java.io.File(destFile); source.setReadable(true); source.setWritable(true,false); source.setExecutable(true,false); try { java.nio.file.Files.copy(source.toPath(), dest.toPath()); oLog.info("Copied"); } catch (java.io.IOException E) { oLog.infoForced("Error"+ E.getMessage()); E.printStackTrace(); } //upload process ParameterPage newParamsPage = new ParameterPage(); newParamsPage.putString("hiddenFileName", fileName); String activityClass = "@baseclass"; String activityName = "pxUploadAndAttachFile"; HashStringMap params = new HashStringMap(); params.putString("pxObjClass", "Rule-Obj-Activity"); params.putString("pyClassName", activityClass); params.putString("pyActivityName", activityName); tools.doActivity(params, myStepPage, newParamsPage); oLog.info("uploaded"); } }
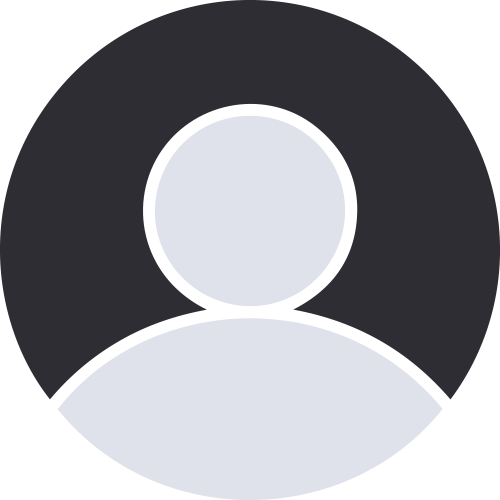
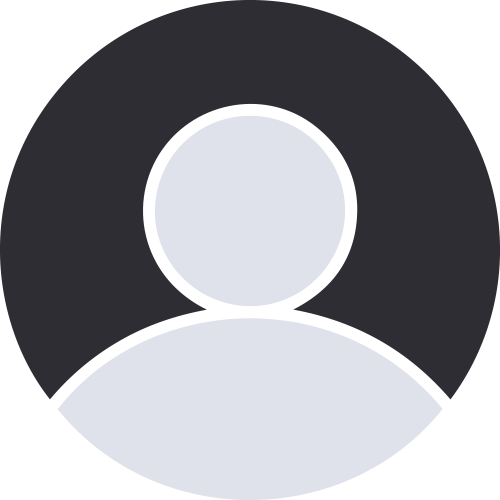
Nationwide Building Society
GB
@TRILOKcan you please share the code to unzip the file
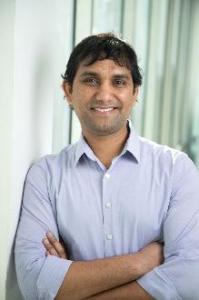
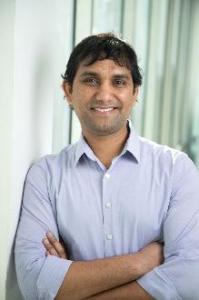
BlueCross BlueShield of NC
US
String fileZip = "/home/web/pega/test.zip"; File destDir = new File("/home/web/pega/dest"); byte[] buffer = new byte[4096]; try { ZipInputStream zis = new ZipInputStream(new FileInputStream(fileZip)); ZipEntry zipEntry = zis.getNextEntry(); while (zipEntry != null) { // File newFile = newFile(destDir, zipEntry); File newFile = new File(destDir, zipEntry.getName()); newFile.setReadable(true); newFile.setWritable(true); newFile.setExecutable(true); if (zipEntry.isDirectory()) { if (!newFile.isDirectory() && !newFile.mkdirs()) { throw new IOException("Failed to create directory " + newFile); } } else { // fix for Windows-created archives File parent = newFile.getParentFile(); if (!parent.isDirectory() && !parent.mkdirs()) { throw new IOException("Failed to create directory " + parent); } // write file content FileOutputStream fos = new FileOutputStream(newFile); int len; while ((len = zis.read(buffer)) > 0) { fos.write(buffer, 0, len); } fos.close(); } zipEntry = zis.getNextEntry(); } zis.closeEntry(); zis.close(); } catch (IOException E) { oLog.infoForced("Error :" + E.getMessage()); }