Question
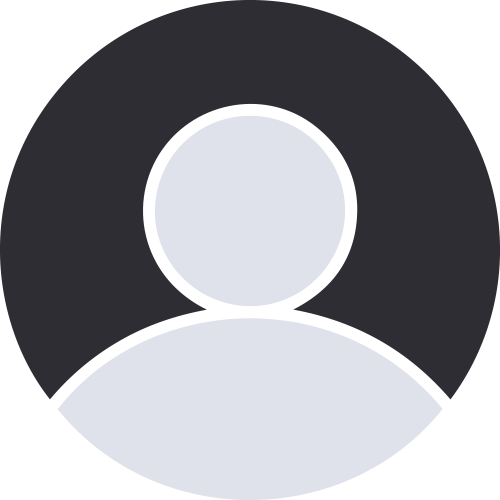
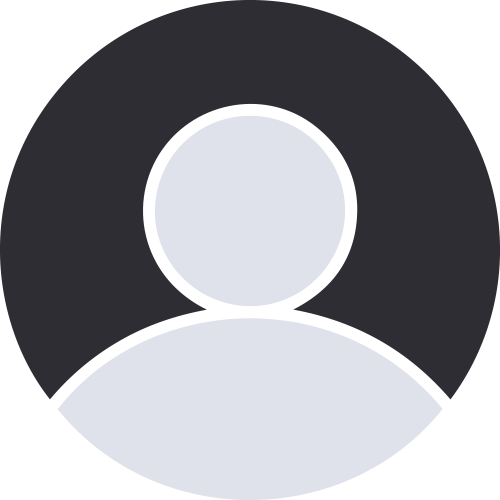
Truviq Systems
IN
Last activity: 14 Mar 2024 13:04 EDT
Merge Word Documents
Hi Guys,
I am looking for a way to merge two word Documents.
Can anyone please help me to write Java Code to merge those documents.
Thanks,
Yaswanth
-
Reply
-
Share this page Facebook Twitter LinkedIn Email Copying... Copied!
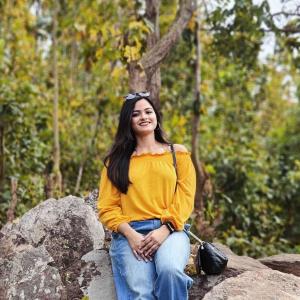
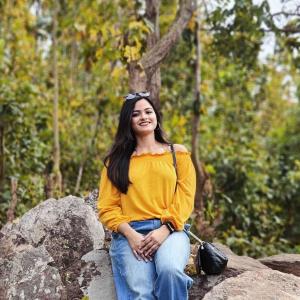
Maantic Technologies Pvt Ltd.
IN
Hi @YaswanthM4584,
You can merge two Word documents using Apache POI (XWPFDocument), assuming you have the base64 encoded content of both documents you may try the below code snippet.
// Decode the base64 strings to byte arrays byte[] doc1Bytes = java.util.Base64.getDecoder().decode(base64Doc1); byte[] doc2Bytes = java.util.Base64.getDecoder().decode(base64Doc2); try{ // Load the documents into XWPFDocument objects org.apache.poi.xwpf.usermodel.XWPFDocument doc1 = new org.apache.poi.xwpf.usermodel.XWPFDocument(new java.io.ByteArrayInputStream(doc1Bytes)); org.apache.poi.xwpf.usermodel.XWPFDocument doc2 = new org.apache.poi.xwpf.usermodel.XWPFDocument(new java.io.ByteArrayInputStream(doc2Bytes));
// Get paragraphs of the second document java.util.List<org.apache.poi.xwpf.usermodel.XWPFParagraph> paragraphs = doc2.getParagraphs();
// Iterate through paragraphs of the second document and add them to the first document for (org.apache.poi.xwpf.usermodel.XWPFParagraph para : paragraphs) { doc1.createParagraph().createRun().setText(para.getText()); }
Hi @YaswanthM4584,
You can merge two Word documents using Apache POI (XWPFDocument), assuming you have the base64 encoded content of both documents you may try the below code snippet.
// Decode the base64 strings to byte arrays byte[] doc1Bytes = java.util.Base64.getDecoder().decode(base64Doc1); byte[] doc2Bytes = java.util.Base64.getDecoder().decode(base64Doc2); try{ // Load the documents into XWPFDocument objects org.apache.poi.xwpf.usermodel.XWPFDocument doc1 = new org.apache.poi.xwpf.usermodel.XWPFDocument(new java.io.ByteArrayInputStream(doc1Bytes)); org.apache.poi.xwpf.usermodel.XWPFDocument doc2 = new org.apache.poi.xwpf.usermodel.XWPFDocument(new java.io.ByteArrayInputStream(doc2Bytes));
// Get paragraphs of the second document java.util.List<org.apache.poi.xwpf.usermodel.XWPFParagraph> paragraphs = doc2.getParagraphs();
// Iterate through paragraphs of the second document and add them to the first document for (org.apache.poi.xwpf.usermodel.XWPFParagraph para : paragraphs) { doc1.createParagraph().createRun().setText(para.getText()); }
// Write the merged document to a file or perform any other operations you need java.io.FileOutputStream fos = new java.io.FileOutputStream("merged_document.docx"); doc1.write(fos); fos.close(); } catch (java.io.IOException e) { oLog.infoForced("Java IO Exception"); }
Updated: 13 Mar 2024 5:45 EDT
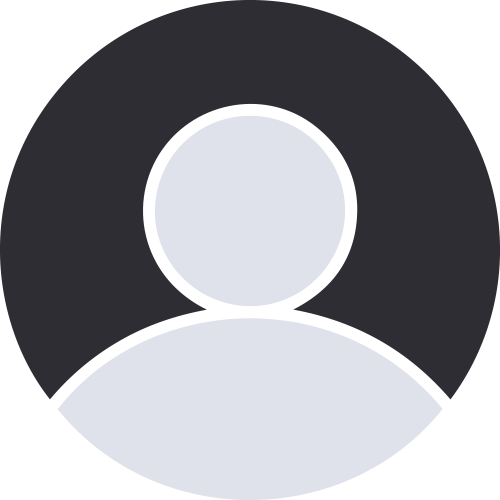
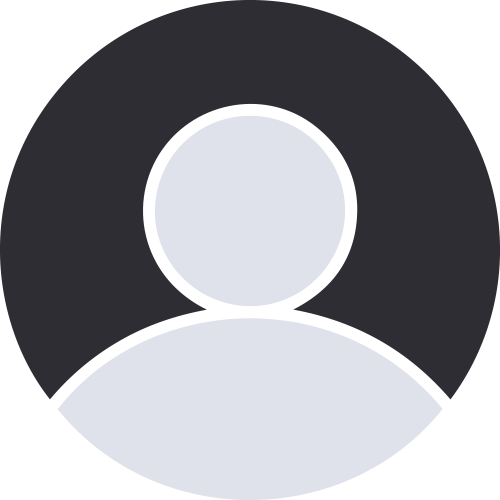
Truviq Systems
IN
@SrijitaB We have tried the above approach and the code compile is successful but not able to generate the document
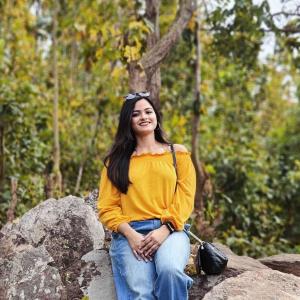
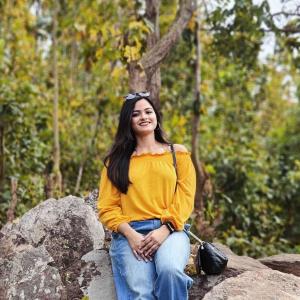
Maantic Technologies Pvt Ltd.
IN
Can you please provide more details? What error are you getting? Are you not able to write the file?
Please note that the code snippet was indicative. Please modify the code as per your needs before executing it.
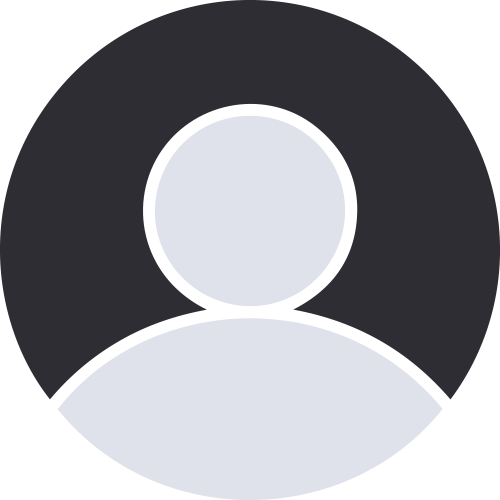
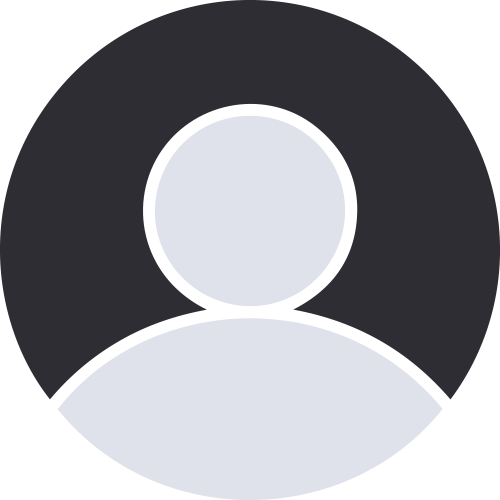
Truviq Systems
IN
@SrijitaB Getting the below error in Stack Trace while executing Java step.
And Could you please provide Jar files which you have imported in Pega and below are the Uploaded Jar files in Pega.
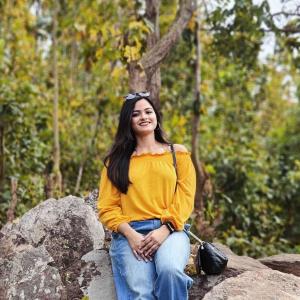
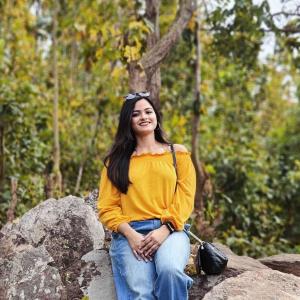
Maantic Technologies Pvt Ltd.
IN
You don't need to import any additional jar file. All the dependent jar files are already available to compile and run the code. If possible can you please share a sample of the documents that you are attempting to merge.
If your document consists of pictures etc. then the above code needs to be modified.
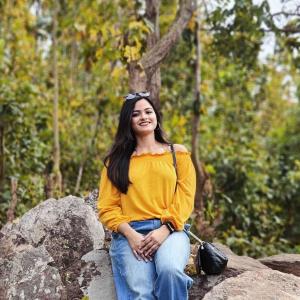
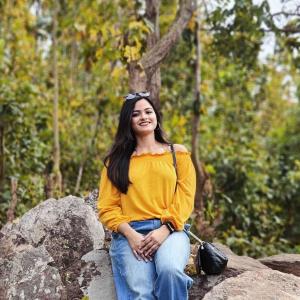
Maantic Technologies Pvt Ltd.
IN
Tried multiple options to merge word documents with embedded images, but was not able to do so successfully. There are some licensed libraries like aspose which can help you to do so, but there are no open source which I could find that can handle such complexity.
Meanwhile if you can get rid of embedded pictures / images I can still help you generate the merged document. Let me know.