Discussion
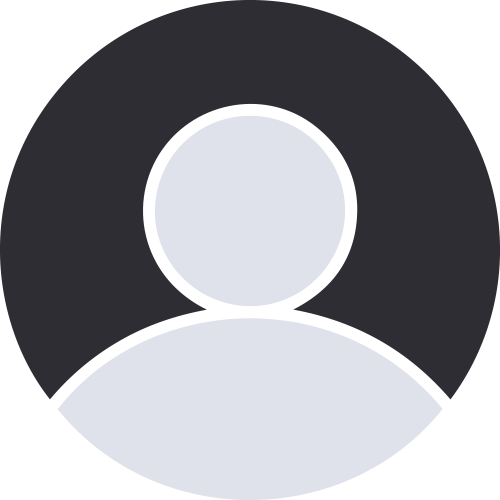
Areteans
Areteans
IN
Areteans
Posted: Jun 28, 2018
Last activity: Feb 3, 2021
Last activity: 3 Feb 2021 14:15 EST
Convert any type of Image to PDF
Hi All,
Below is the code to convert any encoded Base64 Image stream to encoded PDF stream:
It can convert TIFF, JPG, JPEG, PNG, GIF etc.,
//Prepare byte array output stream
java.io.ByteArrayOutputStream outfile = new java.io.ByteArrayOutputStream();
//Create new document
com.lowagie.text.Document document = new com.lowagie.text.Document();
try{
// Get Instance of PDF writer
com.lowagie.text.pdf.PdfWriter writer = com.lowagie.text.pdf.PdfWriter.getInstance(document,outfile);
writer.setStrictImageSequence(true);
// Open the document
document.open();
//TIFF Conversion
if (FileType.equalsIgnoreCase("tif")||FileType.equalsIgnoreCase("tiff"))
{
com.lowagie.text.pdf.RandomAccessFileOrArray ra = new com.lowagie.text.pdf.RandomAccessFileOrArray(Base64Util.decodeToByteArray(attachStream));
// count number of pages in TIFF
int pages = com.lowagie.text.pdf.codec.TiffImage.getNumberOfPages(ra);
for(int i = 0; i< pages;i++)
{
//get each image for TIFF file
com.lowagie.text.Image tiff = com.lowagie.text.pdf.codec.TiffImage.getTiffImage(ra,i+1);
// Scale the Image
float scaler = ((document.getPageSize().getWidth() - document.leftMargin() - document.rightMargin()) / tiff.getWidth()) * 100;
tiff.scalePercent(scaler);
// Add each image to document
document.add(tiff);
}
}
//Other types of images (JPG,JPEG,PNG,GIF etc.,)
else
{
com.lowagie.text.Image jpg = com.lowagie.text.Image.getInstance(Base64Util.decodeToByteArray(attachStream));
//Scale the image
float scaler = ((document.getPageSize().getWidth() - document.leftMargin() - document.rightMargin()) / jpg.getWidth()) * 100;
jpg.scalePercent(scaler);
//Add image to document
document.add(jpg);
}
document.close();
String pdfStream = Base64Util.encodeToString(outfile.toByteArray());
ConvertedStream = pdfStream;
outfile.flush();
}catch(Exception e){
//e.printstacktrace();
}