Discussion
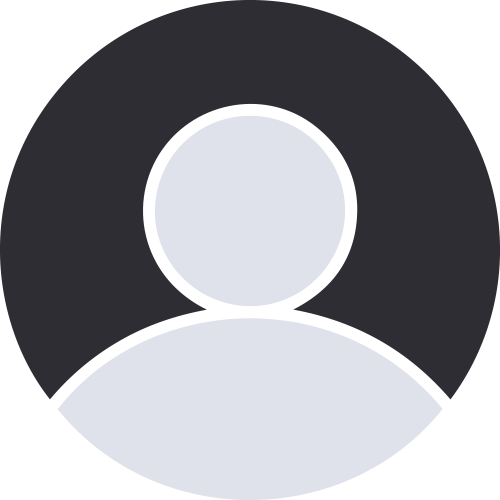
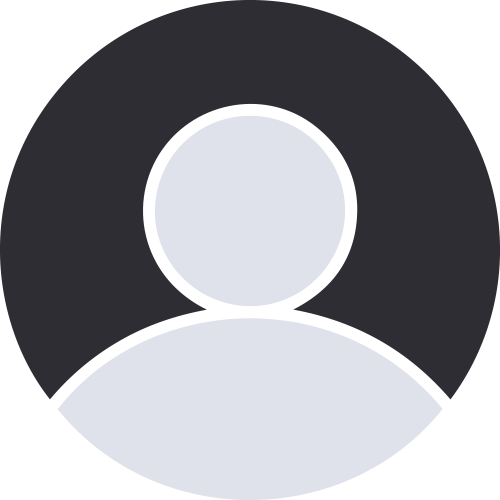
Pegasystems Inc.
US
Last activity: 4 Oct 2018 11:08 EDT
convert clipboard page and/or xml to json
What is the best way to convert a clipboard page and/or xml to json in pega 7.1.5? I imagine there is a java method or pega function that is invoked for REST service functionality... my needs are not REST-specific, but I should be able to use the same assets.
**Moderation Team has archived post**
This post has been archived for educational purposes. Contents and links will no longer be updated. If you have the same/similar question, please write a new post.
-
Like (0)
-
Share this page Facebook Twitter LinkedIn Email Copying... Copied!
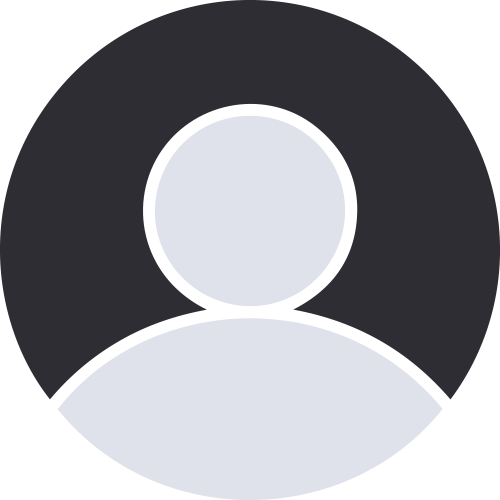
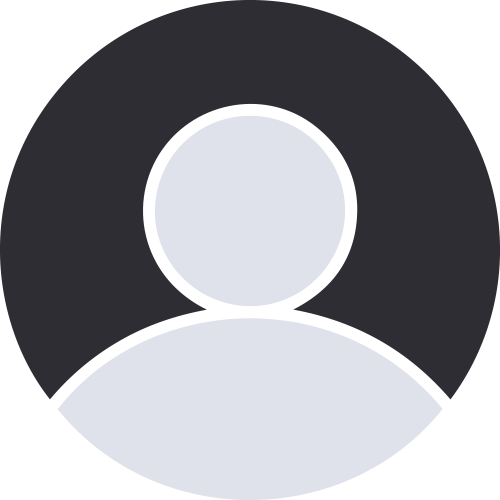
Pegasystems Inc (Test Account 2)
US
The ClipboardPage java API has JSON and XML conversion methods - adoptJSONObject, adoptJSONArray, and adoptXMLForm for populating a clipboard page with JSON/XML data, getJSON and getXML for converting the contents of a clipboard page to an JSON/XML string value.
-
Swathi Palagani
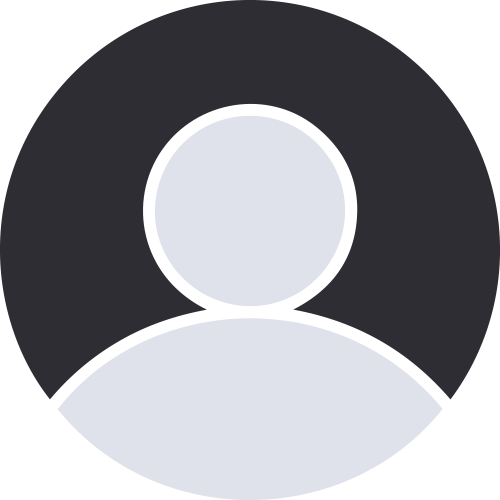
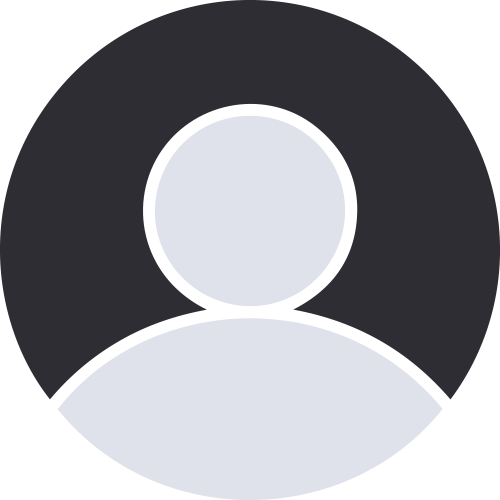
Pegasystems Inc.
US
thanks. that did the trick; i created a simple function that serves my needs:
PublicAPI tools = (PegaAPI) ThreadContainer.get().getPublicAPI();
ClipboardPage stepPage = tools.getStepPage();
String retValue = stepPage.getJSON(false);
return retValue;
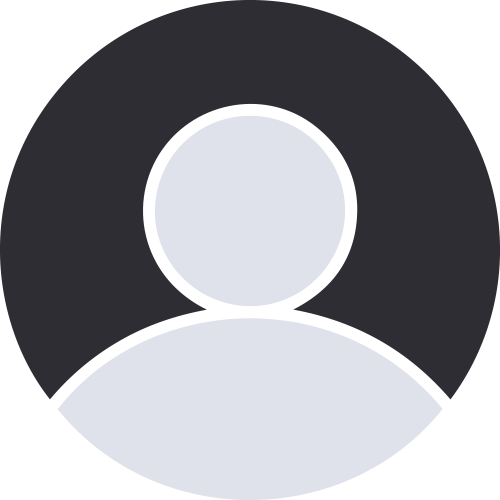
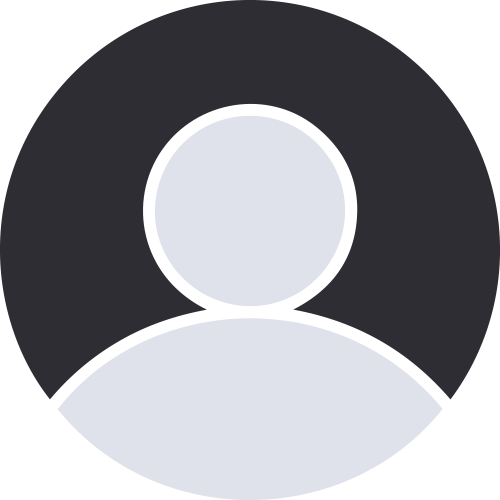
HCL
IN
Hi BONDJ,
My requirement is convert clipboard Page to JSON format.
Can you help me on the same.
Thank You
Ramesh Thimmapur
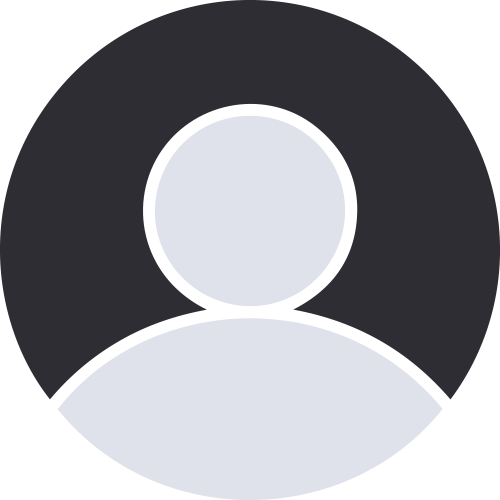
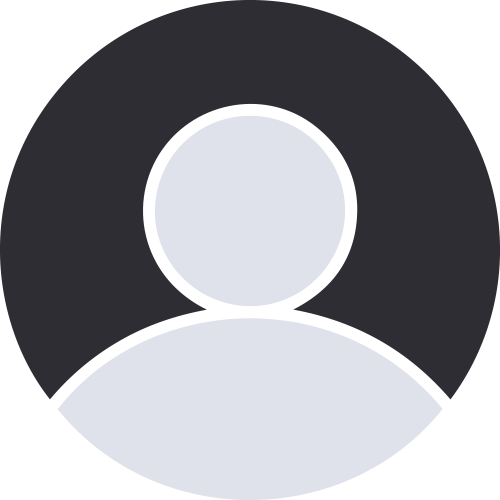
Nielsen
IN
Hi BONDJ,
The above method will also print pxobjclass. Anyway to suppress that?
Regards
Amit
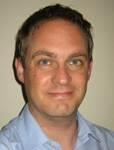
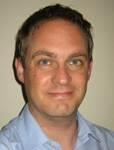
Pegasystems Inc.
GB
This might help:
com.pega.dsm.dnode.util.ClipboardPageJsonConverter converter = new com.pega.dsm.dnode.util.ClipboardPageJsonConverter(false);
net.sf.json.JSONObject json_obj=net.sf.json.JSONObject.fromObject( converter.asJsonString( tools.getStepPage() ) );
net.sf.json.JSONObject stripped_json_obj=new net.sf.json.JSONObject();
Iterator<?> keys = json_obj.keys();
while( keys.hasNext() ) {
String key = (String)keys.next();
if ( (!( key.startsWith("px") )) && (!( key.startsWith("py") )) && (!( key.startsWith("pz") )) ) {
stripped_json_obj.put( key , json_obj.get(key) );
}
}
Or this:
// generate json from this template
com.pega.dsm.dnode.util.ClipboardPageJsonConverter converter = new com.pega.dsm.dnode.util.ClipboardPageJsonConverter(false);
converter.setPrettyPrint(true);
java.nio.ByteBuffer json = converter.asJson(sourcePage);
String jsonString = new String(json.array());
// remove pxObjClass
jsonString = jsonString.replaceAll("\"pxObjClass\"\\s*?:\\s*?\".*?\",?\\s+", "");
return jsonString;
The class/package names may need to altered - depending on your version of PRPC (The first example comes from PRPC72 for instance).
This might help:
com.pega.dsm.dnode.util.ClipboardPageJsonConverter converter = new com.pega.dsm.dnode.util.ClipboardPageJsonConverter(false);
net.sf.json.JSONObject json_obj=net.sf.json.JSONObject.fromObject( converter.asJsonString( tools.getStepPage() ) );
net.sf.json.JSONObject stripped_json_obj=new net.sf.json.JSONObject();
Iterator<?> keys = json_obj.keys();
while( keys.hasNext() ) {
String key = (String)keys.next();
if ( (!( key.startsWith("px") )) && (!( key.startsWith("py") )) && (!( key.startsWith("pz") )) ) {
stripped_json_obj.put( key , json_obj.get(key) );
}
}
Or this:
// generate json from this template
com.pega.dsm.dnode.util.ClipboardPageJsonConverter converter = new com.pega.dsm.dnode.util.ClipboardPageJsonConverter(false);
converter.setPrettyPrint(true);
java.nio.ByteBuffer json = converter.asJson(sourcePage);
String jsonString = new String(json.array());
// remove pxObjClass
jsonString = jsonString.replaceAll("\"pxObjClass\"\\s*?:\\s*?\".*?\",?\\s+", "");
return jsonString;
The class/package names may need to altered - depending on your version of PRPC (The first example comes from PRPC72 for instance).
The JSON handling/conversions in upcoming versions of PRPC will be improved to provide an easier way to perform JSON<->Property mappings; but unfortunately (as far as I know) - you are stuck with having to 'post-process' the JSON as shown above.
-
Ramya Cheera
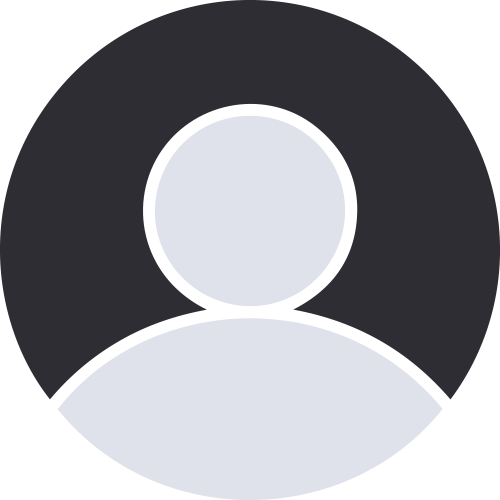
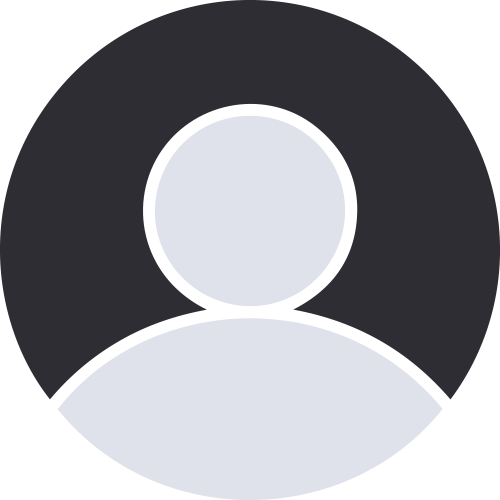
Virtusa Consulting Services
US
We are having issue with the above given snippets..
"net.sf.json cannot be resolved to a type"
what should be the approach?
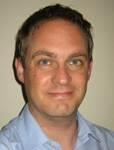
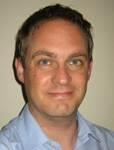
Pegasystems Inc.
GB
What version of PRPC are you using ? It is possible that library I used in the snippet isn't shipped with that version.
If you check 'pr_engineclasses' table - look for anything that has the word 'json' in it as a starting point.
So: something like this:
select distinct(pzjar) from pr_engineclasses
where upper(pzpackage) like '%JSON%'
or upper(pzjar) like '%JSON%'
order by pzjar;
And then basically - see if the JARs are well-known third-party jars, and then check the javadocs for a function that could help.
(it's a bit hacky the above: but that's what I did to find the 'net.sf.json' in the first place!)
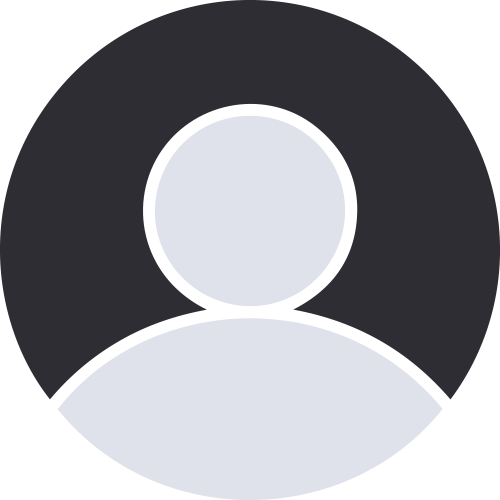
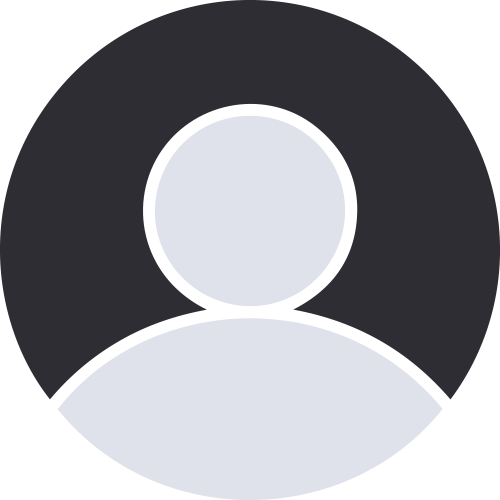
Cognizant
IN
I have simmilar requirement to send JsonObject in Rest Header, I have used the below code to convert Clipboard object to JSON but it gives me a JSON object with pxObjClass. I tried the above approach mentioned but no luck. Please help me
PublicAPI tools = (PegaAPI) ThreadContainer.get().getPublicAPI();
ClipboardPage stepPage = tools.getStepPage();
retValue = stepPage.getJSON(false);