Question
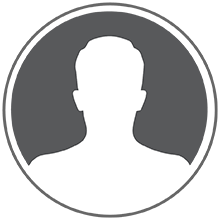
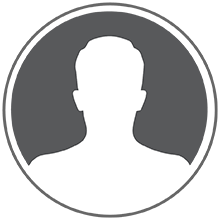
Atos Syntel
IN
Last activity: 1 Sep 2016 5:37 EDT
How to copy a string from a text type property to a date type property
How to copy a string from a text type property to a date type property, say the string is like 2016/05/30.
I have date value (like 2016/05/30) stored in a text type property, i need to copy it to a date type property. Is there any way to do this ?
Message was edited by: Lochan to add Category
-
Like (0)
-
Share this page Facebook Twitter LinkedIn Email Copying... Copied!
Accepted Solution
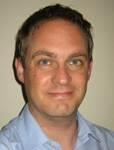
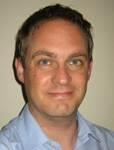
Pegasystems Inc.
GB
[ This is a re-post from a post I made to an internal forum ; but I think it should help here also ]
The following Activity works - using Java Steps.
You might want to re-factor this into a Function (or two functions I guess) : or at least re-factor into a single Activity rather than the two I'm using here (one that using Params, the other using Pages - which calls the first one).
Additionally: I decided to split the Date and Time Components into a PRPC 'Date' Property and a PRPC 'TimeOfDay' Property - this is because these two types have simple well-defined internal-storage formats in the help:
See: (prpchost:port)://prhelp/procomhelpmain.htm#concepts/concepts2/conceptsdatetime.htm
The internal representations
The internal representation of a Date value is eight digits in the format YYYYMMDD, for example 20061215 for December 15, 2006.
The internal representation of Time of Day value is six digits in the format HHMMSS, where the hours portion ranges from 00 to 23. For example, 153025 represents 25 seconds after 3:15 P.M.
Additionally the PRPC help page:
[ This is a re-post from a post I made to an internal forum ; but I think it should help here also ]
The following Activity works - using Java Steps.
You might want to re-factor this into a Function (or two functions I guess) : or at least re-factor into a single Activity rather than the two I'm using here (one that using Params, the other using Pages - which calls the first one).
Additionally: I decided to split the Date and Time Components into a PRPC 'Date' Property and a PRPC 'TimeOfDay' Property - this is because these two types have simple well-defined internal-storage formats in the help:
See: (prpchost:port)://prhelp/procomhelpmain.htm#concepts/concepts2/conceptsdatetime.htm
The internal representations
The internal representation of a Date value is eight digits in the format YYYYMMDD, for example 20061215 for December 15, 2006.
The internal representation of Time of Day value is six digits in the format HHMMSS, where the hours portion ranges from 00 to 23. For example, 153025 represents 25 seconds after 3:15 P.M.
Additionally the PRPC help page:
(prpchost:port)://prhelp/procomhelpmain.htm#designer studio/expressionbuilder/ref_conversions.htm
Has a Table showing different 'casting' operations allowed in Pega7 : and for Text->Date, and Text->TimeOfDay; the casting operation is marked as 'C'.
Which means:
Copies the value without examining whether it is valid.
So it's on us to make sure the Text Property being cast to Date/TimeOfDay is really what we need it to be.
I'm using the Joda Time Library : but this is already included in PRPC - so no need to import anything.
Joda Time has two simple types called 'LocalDate' and 'LocalTime' - which are conceptually the same as a PRPC 'Date' and a PRPC 'TimeOfDay' (They represent just a date and just a time only : no timezones to complicate things).
They also provide validation checks: including rejecting non-existant dates (2015-02-29 will fail [not a leap year], whereas 2016-02-29 is fine [is a leap year]) for instance).
Java Steps are shown below - First Date and then Time:
try {
org.joda.time.LocalDate localdate=new org.joda.time.LocalDate(InDateString);
outDateString=localdate.toString("YYYYMMdd");
}
catch(Exception e) {
throw new PRRuntimeException(e);
}
[NOTE : See my CORRECTION note below for a slight correction to this (the ':' shouldn't be here) - although this step apparently still worked as-is]
try {
org.joda.time.LocalTime localtime=new org.joda.time.LocalTime(InTimeString);
outTimeString = localtime.toString("HH:mm:ss");
}
catch(Exception e) {
throw new PRRuntimeException(e);
}
I have switched on a JUMP step upon exception for both these steps - just jumps to the END of the Activity.
You might want to change this so that it 'dirties' the page rather than chuck an exception - dunno.
[But it's better that it flags the error when it happens rather than finding out later that the date is set to 1970-01-01 I think]
And here's my second testing Activity - which just calls the first: and sets Properties on a Page.
I have three Properties defined on my class here: 'MyInputString' , 'MyDateProp' and 'MyTimeProp'.
They are 'Text', 'Date' and 'TimeOfDay' respectively.
Here's my Page after running it:
<pagedata>
<MyInputString>2016-02-02 13:29:23</MyInputString>
<MyTimeProp>132923</MyTimeProp>
<pxObjClass>Tmp-scratch-Work</pxObjClass>
<MyDateProp>20160202</MyDateProp>
<pzStatus>valid</pzStatus>
</pagedata>
The Activity is essentially hardcoded to only accept dates exactly in one of them variations of the ISO-8601. formats. (Change the Property-Set containing the 'substring' to slice the date /time differently)
CORRECTION:
NOTE: I actually had overly formatted the timestring (although it still appeared to have worked...) in the second Java Step.
The 'internal represenation' for the 'TimeOfDay' type has no separators; so the step should be in fact this:
try {
org.joda.time.LocalTime localtime=new org.joda.time.LocalTime(InTimeString);
outTimeString = localtime.toString("HHmmss");
}
catch(Exception e) {
throw new PRRuntimeException(e);
}
(Whereas it was 'HH:mm:ss')
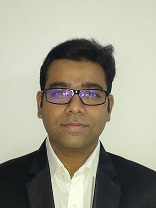
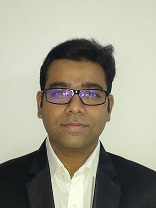
Pegasystems Inc.
IN
Hi
You can try using @ String.toDate(inputString)
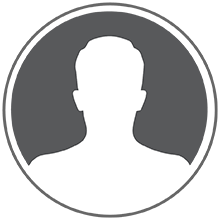
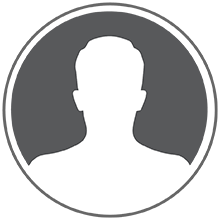
Atos Syntel
IN
Hi Santanu,
I tried your suggestion, @ String.toDate(inputString) returned value 19700101 for string 2016/06/01, i tried changing the date (not the format) it returned the same value (19700101).
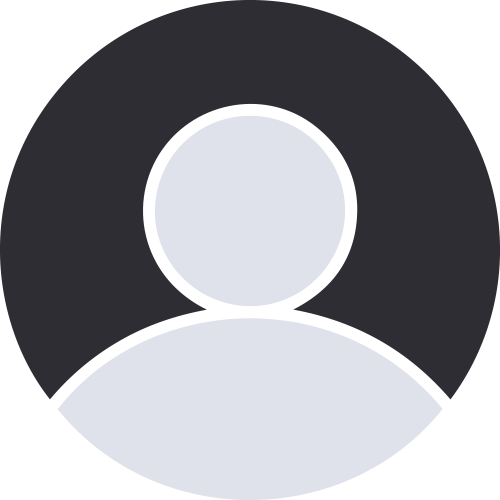
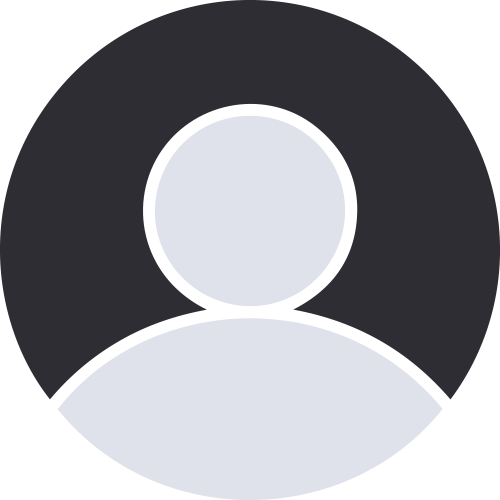
Financial Services
AU
I would probably use FormatDateTime UDF.
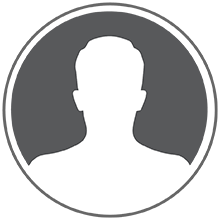
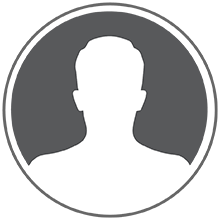
Atos Syntel
IN
i don't think FormatDateTime UDF converts source strings like 2016/06/01, it converts only the standard date time string format (like 20090109T162504.370 GMT) to a required format.
Updated: 29 May 2016 2:25 EDT
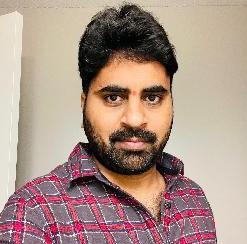
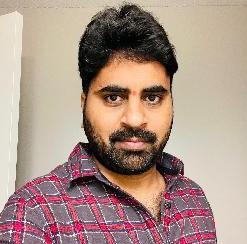
Pegasystems Inc.
US
Hi Venkatesan,
You can write a simple function that takes a String as input parameter and code something like this one:
java.util.Date myDate = new java.text.SimpleDateFormat("yyyy/MM/dd", Locale.ENGLISH).parse(“2016/05/30”);
return myDate;
Kindly let us know if you need further help.
Regards,
Mahesh
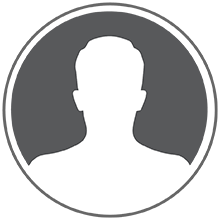
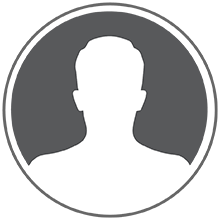
Atos Syntel
IN
tried and got the following error
java.util.Date myDate = new java.text.SimpleDateFormat("yyyy/MM/dd",Locale.ENGLISH).parse("2016/05/30");
^^^^^^
Locale cannot be resolved to a variable
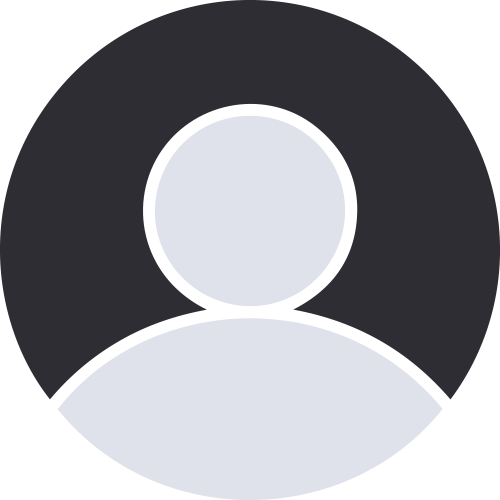
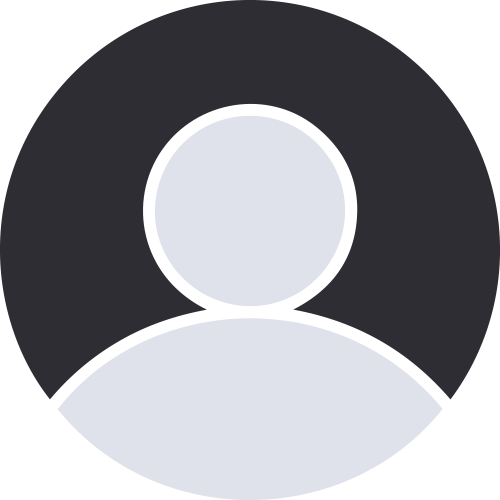
PEG
IN
How about using parseDateTimeStamp and parseDateTimeString
|
parseDateTimeStamp Converts string form of datetimestamp to a Date object. |
|
How about using parseDateTimeStamp and parseDateTimeString
|
parseDateTimeStamp Converts string form of datetimestamp to a Date object. |
|
parseDateTimeString Converts string containing various formats of date & datetime into a Date object. |
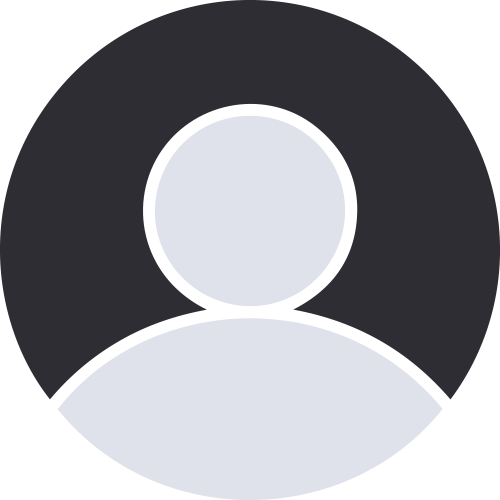
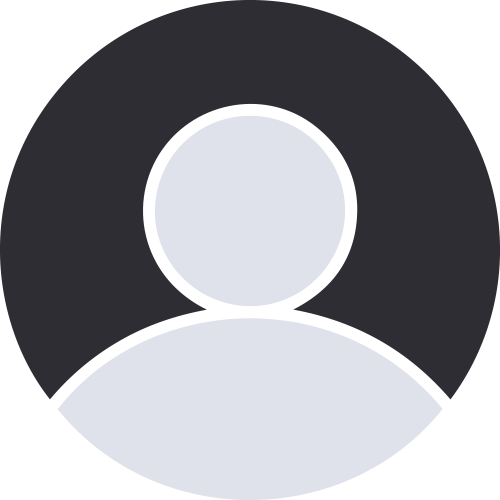
Incessant Technologies
AU
Please check the function "pxFormatDateTime". I was successful using this however, still testing on how the converted value behaves on a report.
Accepted Solution
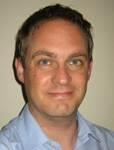
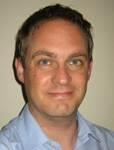
Pegasystems Inc.
GB
[ This is a re-post from a post I made to an internal forum ; but I think it should help here also ]
The following Activity works - using Java Steps.
You might want to re-factor this into a Function (or two functions I guess) : or at least re-factor into a single Activity rather than the two I'm using here (one that using Params, the other using Pages - which calls the first one).
Additionally: I decided to split the Date and Time Components into a PRPC 'Date' Property and a PRPC 'TimeOfDay' Property - this is because these two types have simple well-defined internal-storage formats in the help:
See: (prpchost:port)://prhelp/procomhelpmain.htm#concepts/concepts2/conceptsdatetime.htm
The internal representations
The internal representation of a Date value is eight digits in the format YYYYMMDD, for example 20061215 for December 15, 2006.
The internal representation of Time of Day value is six digits in the format HHMMSS, where the hours portion ranges from 00 to 23. For example, 153025 represents 25 seconds after 3:15 P.M.
Additionally the PRPC help page:
[ This is a re-post from a post I made to an internal forum ; but I think it should help here also ]
The following Activity works - using Java Steps.
You might want to re-factor this into a Function (or two functions I guess) : or at least re-factor into a single Activity rather than the two I'm using here (one that using Params, the other using Pages - which calls the first one).
Additionally: I decided to split the Date and Time Components into a PRPC 'Date' Property and a PRPC 'TimeOfDay' Property - this is because these two types have simple well-defined internal-storage formats in the help:
See: (prpchost:port)://prhelp/procomhelpmain.htm#concepts/concepts2/conceptsdatetime.htm
The internal representations
The internal representation of a Date value is eight digits in the format YYYYMMDD, for example 20061215 for December 15, 2006.
The internal representation of Time of Day value is six digits in the format HHMMSS, where the hours portion ranges from 00 to 23. For example, 153025 represents 25 seconds after 3:15 P.M.
Additionally the PRPC help page:
(prpchost:port)://prhelp/procomhelpmain.htm#designer studio/expressionbuilder/ref_conversions.htm
Has a Table showing different 'casting' operations allowed in Pega7 : and for Text->Date, and Text->TimeOfDay; the casting operation is marked as 'C'.
Which means:
Copies the value without examining whether it is valid.
So it's on us to make sure the Text Property being cast to Date/TimeOfDay is really what we need it to be.
I'm using the Joda Time Library : but this is already included in PRPC - so no need to import anything.
Joda Time has two simple types called 'LocalDate' and 'LocalTime' - which are conceptually the same as a PRPC 'Date' and a PRPC 'TimeOfDay' (They represent just a date and just a time only : no timezones to complicate things).
They also provide validation checks: including rejecting non-existant dates (2015-02-29 will fail [not a leap year], whereas 2016-02-29 is fine [is a leap year]) for instance).
Java Steps are shown below - First Date and then Time:
try {
org.joda.time.LocalDate localdate=new org.joda.time.LocalDate(InDateString);
outDateString=localdate.toString("YYYYMMdd");
}
catch(Exception e) {
throw new PRRuntimeException(e);
}
[NOTE : See my CORRECTION note below for a slight correction to this (the ':' shouldn't be here) - although this step apparently still worked as-is]
try {
org.joda.time.LocalTime localtime=new org.joda.time.LocalTime(InTimeString);
outTimeString = localtime.toString("HH:mm:ss");
}
catch(Exception e) {
throw new PRRuntimeException(e);
}
I have switched on a JUMP step upon exception for both these steps - just jumps to the END of the Activity.
You might want to change this so that it 'dirties' the page rather than chuck an exception - dunno.
[But it's better that it flags the error when it happens rather than finding out later that the date is set to 1970-01-01 I think]
And here's my second testing Activity - which just calls the first: and sets Properties on a Page.
I have three Properties defined on my class here: 'MyInputString' , 'MyDateProp' and 'MyTimeProp'.
They are 'Text', 'Date' and 'TimeOfDay' respectively.
Here's my Page after running it:
<pagedata>
<MyInputString>2016-02-02 13:29:23</MyInputString>
<MyTimeProp>132923</MyTimeProp>
<pxObjClass>Tmp-scratch-Work</pxObjClass>
<MyDateProp>20160202</MyDateProp>
<pzStatus>valid</pzStatus>
</pagedata>
The Activity is essentially hardcoded to only accept dates exactly in one of them variations of the ISO-8601. formats. (Change the Property-Set containing the 'substring' to slice the date /time differently)
CORRECTION:
NOTE: I actually had overly formatted the timestring (although it still appeared to have worked...) in the second Java Step.
The 'internal represenation' for the 'TimeOfDay' type has no separators; so the step should be in fact this:
try {
org.joda.time.LocalTime localtime=new org.joda.time.LocalTime(InTimeString);
outTimeString = localtime.toString("HHmmss");
}
catch(Exception e) {
throw new PRRuntimeException(e);
}
(Whereas it was 'HH:mm:ss')
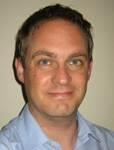
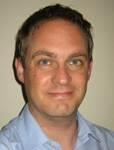
Pegasystems Inc.
GB
Just realized the original post is in fact also available here : https://collaborate.pega.com/question/how-map-string-date-property-data-page